12 min to read
Python - Classes - Methods in classes
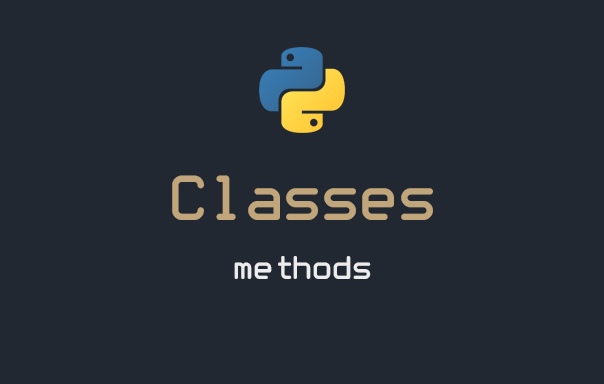
This article is a part of the Python - 101 series, you can access the full version of the series here:
-
Coding 101
-
Writing functions in Python
-
Control flows in Python
-
Errors and exceptions
-
Classes
- Basic
- Methods in classes (you are here!)
Welcome to the twelfth article of the Python - Coding 101 series, which is also the second part of Python Classes. After reading this article, you will learn:
- What is special method in Python
- Most common types of special methods in Python class: __init__, __str__, etc.
In the last article on Python classes - basic, you have learnt how to define classes and methods in classes using class
and def
. There also exists special methods that add magic to your class.
- 1. What is special methods in classes?
- 2. The two most common special methods
- 3. Other important methods in Python
1. What is special methods in classes?
Magic methods are not meant to be invoked directly by you, but the invocation happens internally from the class on a certain action. For example, when you add two numbers using the + operator, internally, the __add__() method will be called.
Built-in classes in Python define many magic methods. Use the dir() function to see the number of magic methods inherited by a class. For example, the following lists all the attributes and methods defined in the int class.
>>> dir(int)
['__abs__', '__add__', '__and__', '__bool__', '__ceil__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floor__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getnewargs__', '__gt__', '__hash__', '__index__', '__init__', '__init_subclass__', '__int__', '__invert__', '__le__', '__lshift__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__or__', '__pos__', '__pow__', '__radd__', '__rand__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rlshift__', '__rmod__', '__rmul__', '__ror__', '__round__', '__rpow__', '__rrshift__', '__rshift__', '__rsub__', '__rtruediv__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', '__xor__', 'bit_length', 'conjugate', 'denominator', 'from_bytes', 'imag', 'numerator', 'real', 'to_bytes']
As you can see above, the int class includes various magic methods surrounded by double underscores. For example, the __add__ method is a magic method which gets called when we add two numbers using the + operator. Consider the following example:
>>> num=10
>>> num + 5
15
>>> num.__add__(5)
15
Magic methods are most frequently used to define overloaded behaviours of predefined operators in Python. For instance, arithmetic operators by default operate upon numeric operands. This means that numeric objects must be used along with operators like +, -, *, /, etc. The + operator is also defined as a concatenation operator in string, list and tuple classes. We can say that the + operator is overloaded.
To prepare to learn more about special methods, first we define a new class called Time:
>>> class Time:
... """Represents the time of day."""
... def print_time(self):
... print('%.2d:%.2d:%.2d' % (self.hour, self.minute, self.second))
2. The two most common special methods
2.1. The __init__ method
The init method (short for “initialization”) is a special method that gets invoked when an object is instantiated. Its full name is __init__ (two underscore characters, followed by init, and then two more underscores). An init method for the Time class might look like this:
>>> # inside class Time:
... def __init__(self, hour=0, minute=0, second=0):
... self.hour = hour
... self.minute = minute
... self.second = second
It is common for the parameters of __init__ to have the same names as the attributes. The statement self.hour = hour stores the value of the parameter hour as an attribute of self. The parameters are optional, so if you call Time with no arguments, you get the default values:
>>> time = Time()
>>> time.print_time()
00:00:00
If you provide one argument, it overrides hour:
>>> time = Time (9)
>>> time.print_time()
09:00:00
If you provide two arguments, they override hour and minute:
>>> time = Time(9, 45)
>>> time.print_time()
09:45:00
And if you provide three arguments, they override all three default values.
2.2. The __str__ method
__str__ is a special method, like __init__, that is supposed to return a string representation of an object.
For example, here is a str method for Time objects:
>>> # inside class Time:
... def __str__(self):
... return '%.2d:%.2d:%.2d' % (self.hour, self.minute, self.second)
When you print an object, Python invokes the str method:
>>> time = Time(9, 45)
>>> print(time)
09:45:00
When I write a new class, I almost always start by writing __init__, which makes it easier to instantiate objects, and __str__, which is useful for debugging.
3. Other important methods in Python
Initialization and Construction | Description |
---|---|
__new__(cls, other) | To get called in an object’s instantiation. |
__init__(self, other) | To get called by the __new__ method. |
__del__(self) | Destructor method. |
Unary operators and functions | Description |
---|---|
__pos__(self) | To get called for unary positive e.g. +someobject. |
__neg__(self) | To get called for unary negative e.g. -someobject. |
__abs__(self) | To get called by built-in abs() function. |
__invert__(self) | To get called for inversion using the ~ operator. |
__round__(self,n) | To get called by built-in round() function. |
__floor__(self) | To get called by built-in math.floor() function. |
__ceil__(self) | To get called by built-in math.ceil() function. |
__trunc__(self) | To get called by built-in math.trunc() function. |
Augmented Assignment | Description |
---|---|
__iadd__(self, other) | To get called on addition with assignment e.g. a +=b. |
__isub__(self, other) | To get called on subtraction with assignment e.g. a -=b. |
__imul__(self, other) | To get called on multiplication with assignment e.g. a *=b. |
__ifloordiv__(self, other) | To get called on integer division with assignment e.g. a //=b. |
__idiv__(self, other) | To get called on division with assignment e.g. a /=b. |
__itruediv__(self, other) | To get called on true division with assignment |
__imod__(self, other) | To get called on modulo with assignment e.g. a%=b. |
__ipow__(self, other) | To get called on exponentswith assignment e.g. a **=b. |
__ilshift__(self, other) | To get called on left bitwise shift with assignment e.g. a«=b. |
__irshift__(self, other) | To get called on right bitwise shift with assignment e.g. a »=b. |
__iand__(self, other) | To get called on bitwise AND with assignment e.g. a&=b. |
__ior__(self, other) | To get called on bitwise OR with assignment e.g. a|=b. |
__ixor__(self, other) | To get called on bitwise XOR with assignment e.g. a ^=b. |
Augmented Assignment | Description |
---|---|
__iadd__(self, other) | To get called on addition with assignment e.g. a +=b. |
__isub__(self, other) | To get called on subtraction with assignment e.g. a -=b. |
__imul__(self, other) | To get called on multiplication with assignment e.g. a *=b. |
__ifloordiv__(self, other) | To get called on integer division with assignment e.g. a //=b. |
__idiv__(self, other) | To get called on division with assignment e.g. a /=b. |
__itruediv__(self, other) | To get called on true division with assignment |
__imod__(self, other) | To get called on modulo with assignment e.g. a%=b. |
__ipow__(self, other) | To get called on exponentswith assignment e.g. a **=b. |
__ilshift__(self, other) | To get called on left bitwise shift with assignment e.g. a«=b. |
__irshift__(self, other) | To get called on right bitwise shift with assignment e.g. a »=b. |
__iand__(self, other) | To get called on bitwise AND with assignment e.g. a&=b. |
__ior__(self, other) | To get called on bitwise OR with assignment e.g. a|=b. |
__ixor__(self, other) | To get called on bitwise XOR with assignment e.g. a ^=b. |
String Magic Methods | Description |
---|---|
__str__(self) | To get called by built-int str() method to return a string representation of a type. |
__repr__(self) | To get called by built-int repr() method to return a machine readable representation of a type. |
__unicode__(self) | To get called by built-int unicode() method to return an unicode string of a type. |
__format__(self, formatstr) | To get called by built-int string.format() method to return a new style of string. |
__hash__(self) | To get called by built-int hash() method to return an integer. |
__nonzero__(self) | To get called by built-int bool() method to return True or False. |
__dir__(self) | To get called by built-int dir() method to return a list of attributes of a class. |
__sizeof__(self) | To get called by built-int sys.getsizeof() method to return the size of an object. |
__ilshift__(self, other) | To get called on left bitwise shift with assignment e.g. a«=b. |
__irshift__(self, other) | To get called on right bitwise shift with assignment e.g. a »=b. |
__iand__(self, other) | To get called on bitwise AND with assignment e.g. a&=b. |
__ior__(self, other) | To get called on bitwise OR with assignment e.g. a|=b. |
__ixor__(self, other) | To get called on bitwise XOR with assignment e.g. a ^=b. |
Attribute Magic Methods | Description |
---|---|
__getattr__(self, name) | Is called when the accessing attribute of a class that does not exist. |
__setattr__(self, name, value) | Is called when assigning a value to the attribute of a class. |
__delattr__(self, name) | Is called when deleting an attribute of a class. |
Operator Magic Methods | Description |
---|---|
__add__(self, other) | To get called on add operation using + operator |
__sub__(self, other) | To get called on subtraction operation using - operator. |
__mul__(self, other) | To get called on multiplication operation using * operator. |
__floordiv__(self, other) | To get called on floor division operation using // operator. |
__div__(self, other) | To get called on division operation using / operator. |
__mod__(self, other) | To get called on modulo operation using % operator. |
__pow__(self, other[, modulo]) | To get called on calculating the power using ** operator. |
__lt__(self, other) | To get called on comparison using < operator. |
__le__(self, other) | To get called on comparison using <= operator. |
__eq__(self, other) | To get called on comparison using == operator. |
__ne__(self, other) | To get called on comparison using != operator. |
__ge__(self, other) | To get called on comparison using >= operator. |
Comments