7 min to read
Python - Control Flows - Conditions
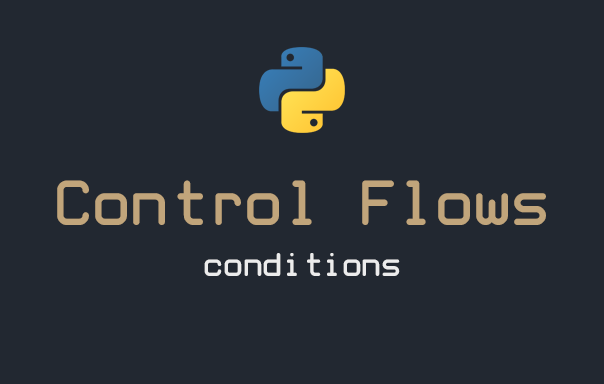
This article is a part of the Python - 101 series, you can access the full version of the series here:
-
Coding 101
-
Writing functions in Python
-
Control flows in Python
- Looping
- Conditions (you are here!)
-
Errors and exceptions
-
Classes
Welcome to the eighth article of the Python - Coding 101 series, after reading this article, you’ll learn:
1. Logical operators
1.1. Comparison operators
1.1.1. Equality
To check if two Python values, or variables, are equal you can use ==
. To check for inequality, you need !=
. As a refresher, have a look at the following examples that all result in True
. Feel free to try them out in the IPython Shell.
2 == (1 + 1)
"intermediate" != "python"
True != False
"Python" != "python"
Note that when you write these comparisons in a script, you will need to wrap a print()
function around them to see the output.
1.1.2. Greater or less than
From the article Python - Coding 101, you must have known about <
and >
in Python. You can combine them with an equals sign: <=
and >=
. Pay attention: <=
is valid syntax, but =<
is not.
All Python expressions in the following code chunk evaluate to True
:
3 < 4
3 <= 4
"alpha" <= "beta"
Remember that for string comparison, Python determines the relationship based on alphabetical order.
1.1.3. Compare array
To compare an array of number, you need to convert them to NumPy array. You can use NumPy array with comparison operators as if it was just a number:
# Create arrays
import numpy as np
my_house = np.array([18.0, 20.0, 10.75, 9.50])
your_house = np.array([14.0, 24.0, 14.25, 9.0])
# my_house greater than or equal to 18
print(my_house >= 18)
# my_house less than your_house
print(my_house < your_house)
1.2. Boolean operator
Now that you can produce boolean by performing comparison operators, the next step is to combine them together
1.2.1. and, or, not with basic data types
A boolean is either 1
or 0
, True
or False
. With boolean operators such as and
, or
and not
, you can combine these booleans to perform more advanced queries on your data.
A rule of thumb to remember is that
-
With
and
, a boolean operator is evaluated to True if and only if every element of the operator is True>>> True and True True >>> True and False False >>> False and True False >>> False and False False
-
With
or
, a boolean operator is evaluated to False if and only if every element of the operator is False>>> True or True True >>> True or False True >>> False or True True >>> False or False False
To evaluate your knowledge so far, give the answer for the question below:
x = 8
y = 9
not(not(x < 3) and not(y > 14 or y > 10))
1.2.2. and, or, not with NumPy array
Before, the operational operators like <
and >=
worked with Numpy arrays out of the box. Unfortunately, this is not true for the boolean operators and
, or
, and not
.
To use these operators with Numpy, you will need np.logical_and()
, np.logical_or()
and np.logical_not()
. Here’s an example on the my_house
and your_house
arrays from before to give you an idea:
# Create arrays
import numpy as np
my_house = np.array([18.0, 20.0, 10.75, 9.50])
your_house = np.array([14.0, 24.0, 14.25, 9.0])
# my_house greater than 18.5 or smaller than 10
print(np.logical_or(my_house > 18.5, my_house < 10))
# Both my_house and your_house smaller than 11
print(np.logical_and(my_house < 11, your_house < 11))
If you insist on using and
instead, Python will yield an error:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
my_house > 18.5 and my_house < 10
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
2. If…elif…else
Depending on the outcome of the comparison, you might want Python to behave differently, that’s when conditional statement comes into play. The syntax is as follow:
if <expr1>:
<statement1>
elif <expr2>:
<statement2>
else:
<statement3>
in which <expr>
is an expression evaluated in Boolean context (give out the result of True or False). The priority of evaluation follow a top-down order, meaning that <expr2>
is evaluated only if <expr1>
is False and <expr3>
is evaluated only if both <expr1>
and <expr2>
is False.
Comments