8 min to read
Python - Errors and Exceptions - Exceptions
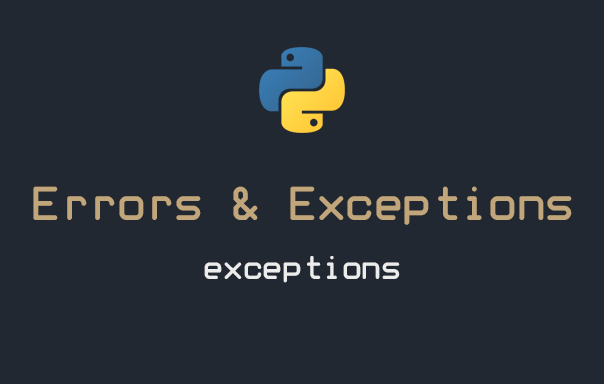
This article is a part of the Python - 101 series, you can access the full version of the series here:
-
Coding 101
-
Writing functions in Python
-
Control flows in Python
-
Errors and exceptions
- Type of errors
- Exceptions (you are here!)
-
Classes
Welcome to the tenth article of the Python - Coding 101 series, which is about Exceptions. After reading this article, you’ll learn:
- What is NameError, TypeError, KeyError, AttributeError, IndexError
- How to use
try...except
The outline of the article is as follow:
In the last article, we have learnt about 3 different types of errors. If you have already read that post, you would remember that we have already mentioned Exceptions. As a quick review, an exception is raised when something goes wrong during runtime. Or in other words, an exception is an event, which occurs during the execution of a program that disrupts the normal flow of the program’s instructions.
There are built-in exceptions, as shown in the figure below, and also user-defined exceptions.

In this article, we will discuss the most common types of exceptions to make you comfort with the feels of encountering exceptions. You will be very likely to see them several times in your coding career, so having knowledge in advance would be beneficial. I will also introduce exceptions handling in the latter part of the post. Exceptions handling makes your code more robust and helps prevent potential failures that would cause your program to stop in an uncontrolled manner.
1. Common types of exceptions
1.1. NameError
You are trying to use a variable that doesn’t exist in the current environment. Check if the name is spelled right, or at least consistently. And remember that local variables are local; you cannot refer to them from outside the function where they are defined.
>>> print(ans)
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
in
----> 1 print(ans)
NameError: name 'ans' is not defined
1.2. TypeError
There are several possible causes:
-
You are trying to use a value improperly. Example: indexing a string, list, or tuple with something other than an integer.
>>> ls = [1,2,3,4,5,6,7] >>> ls['abc'] --------------------------------------------------------------------------- TypeError Traceback (most recent call last) in 1 ls = [1,2,3,4,5,6,7] ----> 2 ls['abc'] TypeError: list indices must be integers or slices, not str
-
There is a mismatch between the items in a format string and the items passed for conversion. This can happen if either the number of items does not match or an invalid conversion is called for.
>>> a = 5 >>> b = "blog by aiden" >>> c = a + b --------------------------------------------------------------------------- TypeError Traceback (most recent call last) in 1 a = 5 2 b = "blog by aiden" ----> 3 c = a + b TypeError: unsupported operand type(s) for +: 'int' and 'str'
-
You are passing the wrong number of arguments to a function. For methods, look at the method definition and check that the first parameter is self. Then look at the method invocation; make sure you are invoking the method on an object with the right type and providing the other arguments correctly.
>>> def bark(sound, times=1): ... print(str(sound) * times) >>> bark(sound='woof', times=2, 3) --------------------------------------------------------------------------- TypeError Traceback (most recent call last) in ----> 1 bark(1,2,3) TypeError: bark() takes from 0 to 2 positional arguments but 3 were given
1.3. KeyError
You are trying to access an element of a dictionary using a key that the dictionary does not contain. If the keys are strings, remember that capitalization matters.
>>> dt = {'key1': 'value1', 'Key2': 'value2'}
>>> dt['key2']
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
in
1 dt = {'key1': 'value1', 'Key2': 'value2'}
----> 2 dt['key2']
KeyError: 'key2'
1.4. IndexError
The index you are using to access a list, string, or tuple is greater than its length minus one.
>>> ls = [1,2,3,4,5,6,7]
>>> ls[100]
---------------------------------------------------------------------------
IndexError Traceback (most recent call last)
in
1 ls = [1,2,3,4,5,6,7]
----> 2 ls[100]
IndexError: list index out of range
To debug this error, immediately before the site of the error, add a print statement to display the value of the index and the length of the array. Is the array the right size? Is the index the right value?
2. Exceptions Handling
As stated in the beginning of the article, exception handling makes your code more robust and helps prevent potential failures that would cause your program to stop in an uncontrolled manner.
Imagine if you have written a code which is deployed in production and still, it terminates due to an exception, your client would not appreciate that, so it’s better to handle the particular exception beforehand and avoid the chaos.
There are four main components of exception handling, as shown below:

As a quick note here, you can use as many except
as you want, an in each except
you can specify as many Exceptions as you want.
Example:
>>> try:
... print (ans)
... except NameError:
... print ("NameError: name 'ans' is not defined")
... except (TypeError, IndexError):
... print ("Other errors occurred)
... else:
... print ("Success, no error!")
NameError: name 'ans' is not defined
In Python programming, exceptions are raised when errors occur at runtime. We can also manually raise exceptions using the raise
keyword.
We can optionally pass values to the exception to clarify why that exception was raised.
The raise
keyword if often used when you want to manage the input to the program.
>>> try:
... a = -1
... if a <= 0:
... raise ValueError("That is not a positive number!")
... except ValueError as ve:
... print(ve)
That is not a positive number!
Comments