6 min to read
Python - Errors and Exceptions - Type of errors
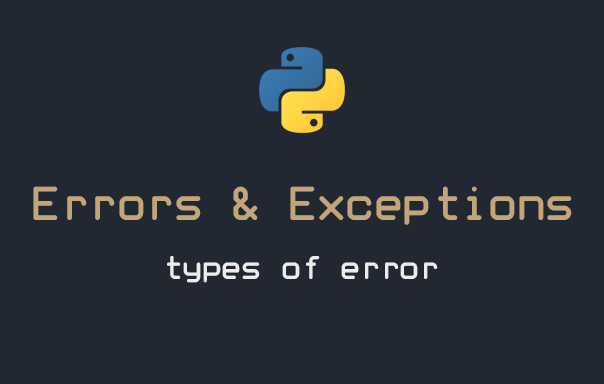
This article is a part of the Python - 101 series, you can access the full version of the series here:
-
Coding 101
-
Writing functions in Python
-
Control flows in Python
-
Errors and exceptions
- Type of errors (you are here!)
- Exceptions
-
Classes
Welcome to the ninth article of the Python - Coding 101 series, which is also the starting of the Errors and Exceptions section. After reading this article, you’ll learn:
- What is syntax errors
- What is runtime errors
- What is semantic errors
- Why do you need to know these types of errors
The outline of the article is as follow:
When writing code, making a mistake is almost inevitable. Such mistakes are called errors in the word of Python.
To understand the important of learning about errors in Python, let’s take this example. Imagine you are doing a test (programming), and you got bad grades (your code do not run, or give out unexpected results) for it, meaning that you made some mistakes (errors) in your answer, but your teacher refuse to tell you where your answer went wrong.
Curious, fidgety? Yeah that’s exactly what errors in programming do to you. Learning about them not only help identify and fix them quickly, but also allow you to avoid them in advance.
1. Different types of errors
Three kinds of errors can occur in a program: syntax errors, runtime errors, and semantic errors. It is useful to distinguish between them in order to track them down more quickly.
1.1. Syntax error
“Syntax” refers to the structure of a program and the rules about that structure. For example, parentheses have to come in matching pairs, so (1 + 2) is legal, but 8) is a syntax error.
If there is a syntax error anywhere in your program, Python displays an error message and quits, and you will not be able to run the program. During the first few weeks of your programming career, you might spend a lot of time tracking down syntax errors. As you gain experience, you will make fewer errors and find them faster.
1.2. Runtime error
The second type of error is a runtime error, so called because the error does not appear until after the program has started running. These errors are also called exceptions because they usually indicate that something exceptional (and bad) has happened.
Runtime errors are rare in the simple programs you will see in the first few chapters, so it might be a while before you encounter one.
1.3. Semantic error
The third type of error is “semantic”, which means related to meaning. If there is a semantic error in your program, it will run without generating error messages, but it will not do the right thing. It will do something else. Specifically, it will do what you told it to do.
Identifying semantic errors can be tricky because it requires you to work backward by looking at the output of the program and trying to figure out what it is doing.
2. How to debug/avoid
2.1. Syntax error
Syntax errors are usually easy to fix once you figure out what they are. Unfortunately,
the error messages are often not helpful. The most common messages are SyntaxEr
ror: invalid syntax
and SyntaxError: invalid token
, neither of which is very
informative.
On the other hand, the message does tell you where in the program the problem occurred. Actually, it tells you where Python noticed a problem, which is not necessarily where the error is. Sometimes the error is prior to the location of the error message, often on the preceding line.
If you are building the program incrementally, you should have a good idea about where the error is. It will be in the last line you added.
If you are copying code from a book, start by comparing your code to the book’s code very carefully. Check every character. At the same time, remember that the book might be wrong, so if you see something that looks like a syntax error, it might be.
2.2. Runtime error
If something goes wrong during runtime, Python prints a message that includes the name of the exception, the line of the program where the problem occurred, and a traceback.
The traceback identifies the function that is currently running, and then the function that called it, and then the function that called that, and so on. In other words, it traces the sequence of function calls that got you to where you are, including the line number in your file where each call occurred.
In fact, the field of exceptions is so wide that it need a whole article on it, which will be discussed in the following article in this 101 series.
2.3. Semantic error
In some ways, semantic errors are the hardest to debug, because the interpreter provides no information about what is wrong. Only you know what the program is supposed to do.
The first step is to make a connection between the program text and the behavior you are seeing. You need a hypothesis about what the program is actually doing. One of the things that makes that hard is that computers run so fast.
You will often wish that you could slow the program down to human speed, and with
some debuggers you can. But personally I would prefer using the print()
statement. The time it takes to insert a few well-placed print statements
is often short compared to setting up the debugger, inserting and removing
breakpoints, and “stepping” the program to where the error is occurring.
To find where your program went wrong, print the value that your variables refer to after each run, especially in loop, where things would scale up very quickly.
Comments